Beta
The pakage is in Beta phase so some bugs occur. If you experience any bugs or have a question then feel free to open an issue.
Qlik Sense Repository Service is in the center of any Qlik Sense cluster. This service is the layer on top of the Repository database and its exposing a quite large set of REST API endpoints.
If we have to manage streams, apps, data connections, virtual proxies, nodes etc. then we have to go through the Repository API endpoints. In the UI world this is all done via the QMC. You can think of QMC as the presentation layer of the Repository API.
qlik-repo-api
NodeJS/browser package exposes number of methods to help developers when interacting with the Repository API.
The installation is simple:
npm install qlik-repo-api
Authentication and initialization
qlik-repo-api
is not performing authentication by itself. But supports multiple authentication mechanisms - certificates, JWT, header, session and ticket. All the authentication information is provided inside the configuration object.
The example below demonstrates how to initialize the client with certificates authentication:
(examples with other authentication methods can be found at the base qlik-rest-api
wiki page)
const https = require("https");
const fs = require("fs");
// read the certificate files
const cert = fs.readFileSync(`/path/to/client.pem`);
const key = fs.readFileSync(`/path/to/client_key.pem`);
// create httpsAgent. Pass the certificate files and ignore any certificate errors (like self-signed certificates)
const httpsAgentCert = new https.Agent({
rejectUnauthorized: false,
cert: cert,
key: key,
});
// the actual initialization
const repoApi = new QlikRepoApi.client({
host: "my-sense-server.com",
port: 4242, // optional. default 4242
httpsAgent: httpsAgentCert,
authentication: {
user_dir: "USER-DIRECTORY",
user_name: "userId",
},
});
Usage
At this point we've just initialized the client and no communication with Qlik is made.
To start the actual usage let's get list of apps:
const filterApp = await repoApi.apps.getFilter({
filter: `name sw 'License'`,
});
At this point filterApp
will contain an array of app instances. Being an app instance means that we can perform operations on each element:
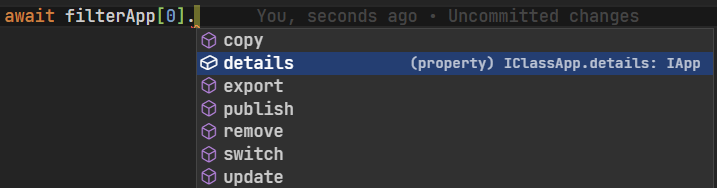
All the app metadata is under details
:
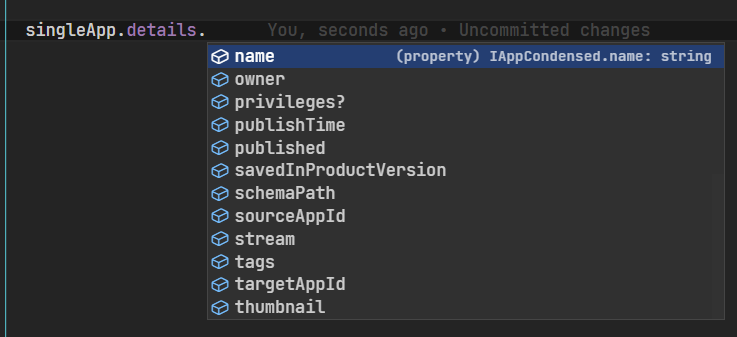
And, for example, if we want to update all the apps from the filter by adding custom properties we can use:
const updateResults = await Promise.all(
filterApp.map((app) =>
app.update({
customProperties: [
"customProperty1=value1",
"customProperty1=value2",
"customProperty2=some-value",
],
})
)
);
And because we are operating on the app instances, once the update is performed the values in our filterApp
array will also be updated.
qlik-repo-api
also exposes types and this plays nice with the IDEs. For example if we have to call update()
method on an app instance, then the IDE's intellisense will show us what parameters should be provided and if they are optional or not:
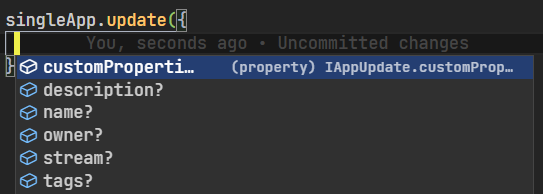
And more ...
To know more about the pakage, please use the links below